obj
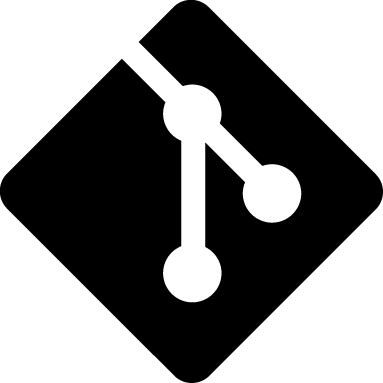
Vars | |
attacked_verb | Verb used when the object is punched. If defined, overrides the punch's usual verb |
---|---|
buckle_pixel_shift | A list of (x, y, z) to offset buckled_mob by, or null. Best assigned to reference a static list, eg: /myobj var/static/list/myobj_buckle_pixel_shift = list(0, 0, 6) /myobj/Destroy() buckle_pixel_shift = null /myobj/Initialize() buckle_pixel_shift = myobj_buckle_pixel_shift |
edge | Whether this object is more likely to dismember |
puncture | For items that can puncture e.g. thick plastic but aren't necessarily sharp. Called in can_puncture() |
sharp | whether this object cuts |
Procs | |
AttemptBuckle | Attempts to buckle the target to the object. Includes can_buckle() checks and a timer. Calls user_buckle_mob() . |
AttemptUnbuckle | Attempts to unbuckle the object's buckled mob. Includes can_unbuckle() checks and a timer. Calls user_unbuckle_mob() . |
SetupChameleonExtension | Call this proc to automatically setup the best suited chameleon extension for the instance, if one exists. |
buckle_mob | Handles buckling the given mob. Assumes most conditions are met - This only checks if loc is the same and if the mob's buckled is null to avoid broken states. |
can_anchor | Whether or not the object can be anchored in its current state/position. Assumes the anchorable flag has already been checked. |
can_buckle | Checks if a mob can be buckled to the object. |
can_unbuckle | Checks if a mob can unbuckle the object. |
clear_buckle | Clears any buckling references that exist between object and buckled mob, without clearing any unrelated references. Used by the destroyed event handler. |
is_safe_to_step | Test for if stepping on a tile containing this obj is safe to do, used for things like landmines and cliffs. |
post_anchor_change | Called when the object's anchor state is changed via wrench_floor_bolts() . |
unbuckle_mob | Handles unbuckling any buckled mobs. Assumes all conditions are met. |
user_buckle_mob | Handles buckling a mob by another mob (Or the same mob). Includes can_buckle() checks. |
user_unbuckle_mob | Handles unbuckling a mob by another mob (Or the same mob). Includes can_unbuckle() checks. |
Var Details
attacked_verb
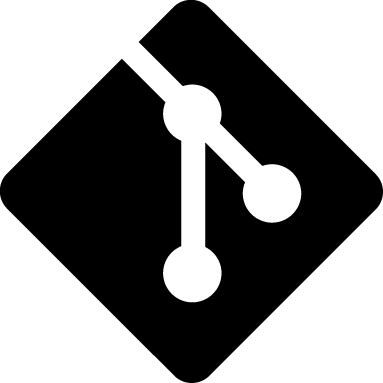
Verb used when the object is punched. If defined, overrides the punch's usual verb
buckle_pixel_shift
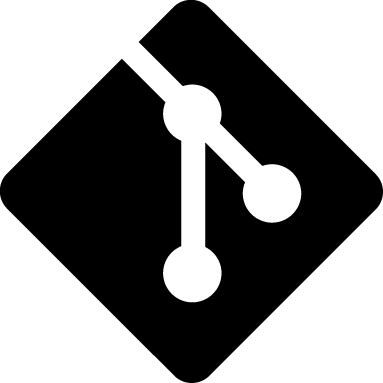
A list of (x, y, z) to offset buckled_mob by, or null. Best assigned to reference a static list, eg: /myobj var/static/list/myobj_buckle_pixel_shift = list(0, 0, 6) /myobj/Destroy() buckle_pixel_shift = null /myobj/Initialize() buckle_pixel_shift = myobj_buckle_pixel_shift
edge
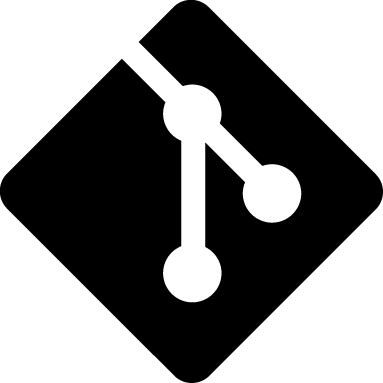
Whether this object is more likely to dismember
puncture
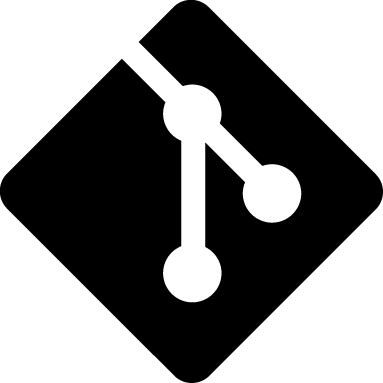
For items that can puncture e.g. thick plastic but aren't necessarily sharp. Called in can_puncture()
sharp
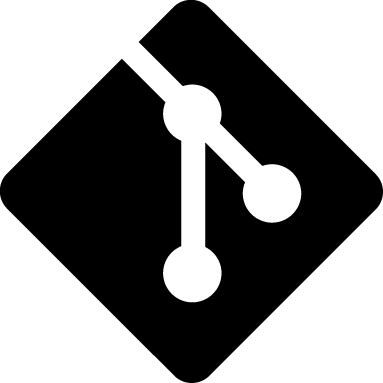
whether this object cuts
Proc Details
AttemptBuckle
Attempts to buckle the target to the object. Includes can_buckle()
checks and a timer. Calls user_buckle_mob()
.
Generally, you should call this during user interactions instead of directly calling the buckle procs.
Parameters:
target
- Mob to be buckled.user
- Mob attempting to perform the buckling. Target of failure feedback messages.silent
(Boolean, defaultFALSE
) - If set, does not display feedback messages. Passed tocan_buckle()
anduser_buckle_mob()
.
Returns boolean. Whether or not the buckling was successful.
AttemptUnbuckle
Attempts to unbuckle the object's buckled mob. Includes can_unbuckle()
checks and a timer. Calls user_unbuckle_mob()
.
Generally, you should call this during user interactions instead of directly calling the buckle procs.
Parameters:
user
- Mob attempting to perform the unbuckling. Target of failure feedback messages.silent
(Boolean, defaultFALSE
) - If set, does not display feedback messages. Passed tocan_unbuckle()
anduser_unbuckle_mob()
.
Returns boolean. Whether or not the buckling was successful.
SetupChameleonExtension
Call this proc to automatically setup the best suited chameleon extension for the instance, if one exists.
Exceptions:
- If the instance only matches the base /datum/extension/chameleon type it is not set for performance reasons. For these
set_extension()
has to be called explicitly. - If the instance already has the /datum/extension/chameleon extension it is not overriden, but the proc still returns
TRUE
.
Parameters:
chamelon_options
- Based on the relevant CHAMELEON_FLEXIBLE_OPTION_* argumentexclude_outfits
- Whether to exclude the chameleon outfit verbs.throw_runtime
- Whether to throw a runtime exception if no matching extension was found. This includes cases when /datum/extension/chameleon would've been a match had it not been for its exclusion.
Returns boolean - Whether or not a matching extension was found
buckle_mob
Handles buckling the given mob. Assumes most conditions are met - This only checks if loc
is the same and if the mob's buckled
is null to avoid broken states.
Returns boolen - Whether or not the mob was buckled.
can_anchor
Whether or not the object can be anchored in its current state/position. Assumes the anchorable flag has already been checked.
Parameters:
tool
- Tool being used to un/anchor the object.user
- User performing the interaction.silent
(Boolean, defaultFALSE
) - If set, does not send user feedback messages on failure.
Returns boolean.
can_buckle
Checks if a mob can be buckled to the object.
Parameters:
target
- Mob to be buckled.user
- Optional. Mob attempting to perform the buckling. Target of failure feedback messages. If not provided, checks requiringuser
are not performed.silent
(Boolean, defaultFALSE
) - If set, does not send failure feedback messages touser
.
Returns boolean.
can_unbuckle
Checks if a mob can unbuckle the object.
Parameters:
user
- Optional. Mob attempting to perform the unbuckling. Target of failure feedback messages. If not provided, checks onuser
are not performed.silent
(Boolean, defaultFALSE
) - If set, does not send failure feedback messages touser
.
Returns boolean.
clear_buckle
Clears any buckling references that exist between object and buckled mob, without clearing any unrelated references. Used by the destroyed event handler.
Includes some redundancy to ensure all references are clear.
is_safe_to_step
Test for if stepping on a tile containing this obj is safe to do, used for things like landmines and cliffs.
post_anchor_change
Called when the object's anchor state is changed via wrench_floor_bolts()
.
unbuckle_mob
Handles unbuckling any buckled mobs. Assumes all conditions are met.
Returns a reference to the previously buckled mob, or null.
user_buckle_mob
Handles buckling a mob by another mob (Or the same mob). Includes can_buckle()
checks.
Generally, you should be calling AttemptBuckle()
instead of this.
Parameters:
target
- Mob to be buckled.user
- Mob attempting to perform the buckling. Target of failure feedback messages.silent
(Boolean, defaultFALSE
) - If set, does not display feedback messages. Passed tocan_buckle()
.
Returns boolean. Whether or not the buckling was successful.
user_unbuckle_mob
Handles unbuckling a mob by another mob (Or the same mob). Includes can_unbuckle()
checks.
Generally, you should be calling AttemptUnbuckle()
instead of this.
Parameters:
user
- Mob attempting to perform the unbuckling. Target of failure feedback messages.silent
(Boolean, defaultFALSE
) - If set, does not display feedback messages. Passed tocan_unbuckle()
.
Returns instance of the unbuckled mob or null on failure.